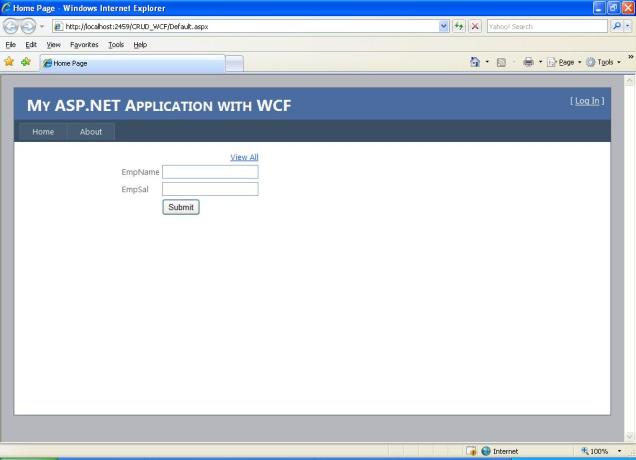
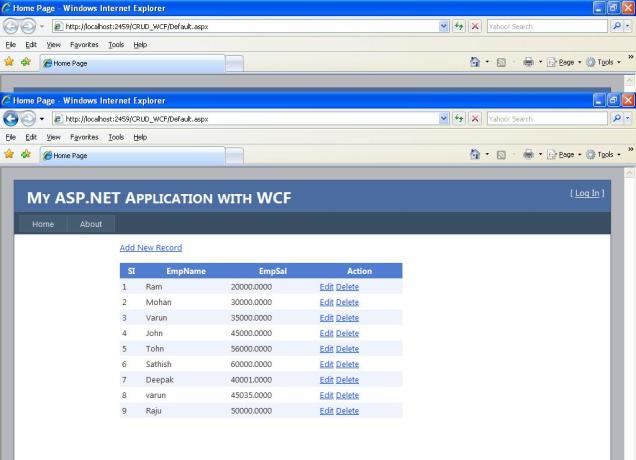
Hi
I hope that you are know the basic concept of creating and consuming WCF. If you don’t know then please refer my previous post
Wcf sample-code in asp.net 3.5.
For simplicity i have written General sql query. Please change into store procedure for better performance.
For doing this task, we have to do like this
Step1:Write the code in “Iservice.cs” like this
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
// NOTE: If you change the interface name “IService2” here, you must also update the reference to “IService2” in Web.config.
[ServiceContract]
public interface IService
{
[OperationContract]
List<Emp> GetAllEmpName();
[OperationContract]
int SaveEmp(Emp objEmp);
[OperationContract]
int DeleteEmp(int Id);
[OperationContract]
List<Emp> GetSelectedEmp(int Id);
[OperationContract]
int UpdateSelectedEmp(Emp objEmp);
}
[DataContract]
public class Emp
{
[DataMember]
public int Id { get; set; }
[DataMember]
public string EmpName { get; set; }
[DataMember]
public string EmpSal { get; set; }
}
Step2 write the code in “Service.cs” like this
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
using System.Data;
using System.Data.SqlClient;
// NOTE: If you change the class name “Service2” here, you must also update the reference to “Service2” in Web.config.
public class Service : IService
{
public void DoWork()
{
}
//For Fetching All EmpName
public List<Emp> GetAllEmpName()
{
List<Emp> Emps = new List<Emp>();
using (SqlConnection con = new SqlConnection(“Data Source=.\\SQLEXPRESS;Initial Catalog=Test;Integrated Security=True”))
{
using (SqlCommand cmd = new SqlCommand(“Select Id,EmpName,EmpSal from tblEmp”, con))
{
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
while (dr.Read())
{
Emp emp1 = new Emp();
emp1.Id = int.Parse(dr[“Id”].ToString());
emp1.EmpName = Convert.ToString(dr[“EmpName”]);
emp1.EmpSal = Convert.ToString(dr[“EmpSal”]);
Emps.Add(emp1);
}
con.Close();
return Emps;
}
}
}
//For saving Emp Detail
public int SaveEmp(Emp objEmp)
{
using (SqlConnection con = new SqlConnection(“Data Source=.\\SQLEXPRESS;Initial Catalog=Test;Integrated Security=True”))
{
using (SqlCommand cmd = new SqlCommand(“Insert into tblEmp(EmpName,EmpSal)Values(@EmpName,@EmpSal)”, con))
{
cmd.Parameters.AddWithValue(“@EmpName”, objEmp.EmpName);
cmd.Parameters.AddWithValue(“@EmpSal”, objEmp.EmpSal);
con.Open();
int a = cmd.ExecuteNonQuery();
con.Close();
return a;
}
}
}
//For Deleting Emp Record
public int DeleteEmp(int Id)
{
using (SqlConnection con = new SqlConnection(“Data Source=.\\SQLEXPRESS;Initial Catalog=Test;Integrated Security=True”))
{
using (SqlCommand cmd = new SqlCommand(“Delete from tblEmp where Id=@Id”, con))
{
cmd.Parameters.AddWithValue(“@Id”, Id);
con.Open();
int a = cmd.ExecuteNonQuery();
con.Close();
return a;
}
}
}
//For Updating Emp Detail
public int UpdateSelectedEmp(Emp objEmp)
{
using (SqlConnection con = new SqlConnection(“Data Source=.\\SQLEXPRESS;Initial Catalog=Test;Integrated Security=True”))
{
using (SqlCommand cmd = new SqlCommand(“Update tblEmp set EmpName=@EmpName,EmpSal=@EmpSal where Id=@Id”, con))
{
cmd.Parameters.AddWithValue(“@Id”, objEmp.Id);
cmd.Parameters.AddWithValue(“@EmpName”, objEmp.EmpName);
cmd.Parameters.AddWithValue(“@EmpSal”, objEmp.EmpSal);
con.Open();
int a = cmd.ExecuteNonQuery();
con.Close();
return a;
}
}
}
//For Fetching Particular EmpDetail
public List<Emp> GetSelectedEmp(int Id)
{
List<Emp> Emps = new List<Emp>();
using (SqlConnection con = new SqlConnection(“Data Source=.\\SQLEXPRESS;Initial Catalog=Test;Integrated Security=True”))
{
using (SqlCommand cmd = new SqlCommand(“Select *from tblEmp where Id=@Id”, con))
{
con.Open();
cmd.Parameters.AddWithValue(“@Id”, Id);
SqlDataReader dr = cmd.ExecuteReader();
while (dr.Read())
{
Emp emp1 = new Emp();
emp1.Id = int.Parse(dr[“Id”].ToString());
emp1.EmpName = Convert.ToString(dr[“EmpName”]);
emp1.EmpSal = Convert.ToString(dr[“EmpSal”]);
Emps.Add(emp1);
}
con.Close();
return Emps;
}
}
}
}
Step3: Design default page like this
<div style=”margin-left:150px”>
<asp:MultiView ID=”Mv1″ runat=”server”>
<asp:View ID=”Vw1″ runat=”server”>
<table>
<tr>
<td>
</td>
<td align=”right”>
<asp:LinkButton ID=”LinkButton1″ runat=”server” onclick=”LinkButton1_Click”
Width=”88px”>View All</asp:LinkButton>
</td>
<td>
</td>
</tr>
<tr>
<td>
EmpName</td>
<td>
<asp:TextBox ID=”txtEmpName” runat=”server”></asp:TextBox>
</td>
<td>
</td>
</tr>
<tr>
<td>
EmpSal</td>
<td>
<asp:TextBox ID=”txtEmpSal” runat=”server”></asp:TextBox>
</td>
<td>
</td>
</tr>
<tr>
<td>
</td>
<td>
<asp:Button ID=”BtnSubmit” runat=”server” Text=”Submit”
onclick=”BtnSubmit_Click” style=”height: 26px” />
</td>
<td>
</td>
</tr>
<tr>
<td>
</td>
<td>
<asp:Label ID=”lblmsg” runat=”server”></asp:Label>
</td>
<td>
</td>
</tr>
<tr>
<td>
</td>
<td>
<asp:HiddenField ID=”HiddenField1″ runat=”server” />
</td>
<td>
</td>
</tr>
</table>
</asp:View>
<asp:View ID=”Vw2″ runat=”server”>
<asp:LinkButton ID=”lnkBack” Text=”Add New Record” runat=”server”
onclick=”lnkBack_Click” /><br /><br />
<asp:GridView ID=”GridView1″ AllowPaging=”true” PageSize=”10″ runat=”server” AutoGenerateColumns=”False”
CellPadding=”4″ ForeColor=”#333333″ GridLines=”None”
onpageindexchanging=”GridView1_PageIndexChanging”
onrowcommand=”GridView1_RowCommand” onrowdeleting=”GridView1_RowDeleting”
onrowediting=”GridView1_RowEditing” Width=”455px”>
<RowStyle BackColor=”#EFF3FB” />
<Columns>
<asp:TemplateField HeaderText=”SI”>
<ItemTemplate>
<%#Container.DataItemIndex+1 %>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField HeaderText=”EmpName” DataField=”EmpName” />
<asp:BoundField HeaderText=”EmpSal” DataField=”EmpSal” />
<asp:TemplateField HeaderText=”Action”>
<ItemTemplate>
<asp:LinkButton ID=”lnkEdit” runat=”server” Text=”Edit” CausesValidation=”false” CommandName=”Edit” CommandArgument='<%# Eval(“Id”) %>’ OnClientClick=”return confirm(‘Are you sure?’)” />
<asp:LinkButton runat=”server” ID=”lnk_Delete” Text=”Delete” CausesValidation=”false” CommandName=”Delete” CommandArgument='<%# Eval(“Id”) %>’ OnClientClick=”return confirm(‘Are you sure?’)”/>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor=”#507CD1″ Font-Bold=”True” ForeColor=”White” />
<PagerStyle BackColor=”#2461BF” ForeColor=”White” HorizontalAlign=”Center” />
<SelectedRowStyle BackColor=”#D1DDF1″ Font-Bold=”True” ForeColor=”#333333″ />
<HeaderStyle BackColor=”#507CD1″ Font-Bold=”True” ForeColor=”White” />
<EditRowStyle BackColor=”#2461BF” />
<AlternatingRowStyle BackColor=”White” />
</asp:GridView>
</asp:View>
</asp:MultiView>
<br />
</div>
step 4: write the code in code behind file like this
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
Mv1.ActiveViewIndex = 0;
}
}
protected void GridView1_PageIndexChanging(object sender, GridViewPageEventArgs e)
{
//this is used for paging in gridview
GridView1.PageIndex = e.NewPageIndex;
fillGrid();
}
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == “Delete”)
{
//This is used for deleting operation
int id1 = Convert.ToInt32(e.CommandArgument);
using (ShowEmpData.ServiceClient ws = new ShowEmpData.ServiceClient())
{
int a = ws.DeleteEmp(id1);
if (a == 1)
{
fillGrid();
Mv1.ActiveViewIndex = 1;
}
}
}
else if (e.CommandName == “Edit”)
{
//this is used for displaying particular record for updation work
int id1 = Convert.ToInt32(e.CommandArgument);
using (ShowEmpData.ServiceClient ws = new ShowEmpData.ServiceClient())
{
var Empobj = ws.GetSelectedEmp(id1);
txtEmpName.Text = Empobj[0].EmpName.ToString();
txtEmpSal.Text = Empobj[0].EmpSal.ToString();
HiddenField1.Value = Empobj[0].Id.ToString();
BtnSubmit.Text = “Update”;
lblmsg.Text = “”;
Mv1.ActiveViewIndex = 0;
}
}
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
}
//This is used for filling gridview
protected void fillGrid()
{
ShowEmpData.ServiceClient ws = new ShowEmpData.ServiceClient();
GridView1.DataSource = ws.GetAllEmpName();
GridView1.DataBind();
}
protected void BtnSubmit_Click(object sender, EventArgs e)
{
if (BtnSubmit.Text == “Submit”)
{
using (ShowEmpData.ServiceClient ws = new ShowEmpData.ServiceClient())
{
// This is used for Insertion of records
ShowEmpData.Emp objemp = new ShowEmpData.Emp();
objemp.EmpName = txtEmpName.Text;
objemp.EmpSal = txtEmpSal.Text;
int a = ws.SaveEmp(objemp);
if (a == 1)
{
txtEmpName.Text = “”;
txtEmpSal.Text = “”;
fillGrid();
Mv1.ActiveViewIndex = 1;
}
}
}
else
{
// This is used for updating the records
using (ShowEmpData.ServiceClient ws = new ShowEmpData.ServiceClient())
{
ShowEmpData.Emp objemp = new ShowEmpData.Emp();
objemp.Id = Convert.ToInt32(HiddenField1.Value);
objemp.EmpName = txtEmpName.Text;
objemp.EmpSal = txtEmpSal.Text;
int a = ws.UpdateSelectedEmp(objemp);
if (a == 1)
{
txtEmpName.Text = “”;
txtEmpSal.Text = “”;
fillGrid();
Mv1.ActiveViewIndex = 1;
}
}
}
}
protected void LinkButton1_Click(object sender, EventArgs e)
{
Mv1.ActiveViewIndex = 1;
fillGrid();
}
protected void lnkBack_Click(object sender, EventArgs e)
{
Mv1.ActiveViewIndex = 0;
BtnSubmit.Text = “Submit”;
}
}
12.971599
77.594563